What are functions in python and how do they work?
An introduction to functions and how they work.
I have a question for you: what's the capital of Spain? ... Hmm? Oh, right! Madrid. I forgot that, thanks. ...hey, but since you're already here, I need your help with something: what's the capital of Spain? Oh, that's right.... Madrid. Thanks! Hey, one last question before you go: what's the capital of Spain? ...
In the preceding paragraph, I asked the same question a few times and it got tiresome real fast, right? You already provided an answer the first time, so it probably seemed a bit silly to be continually asked for the same thing and be expected to put forth the same effort to derive the answer. That belief is absolutely true for programming as well – duplicating effort (writing excess code) is inefficient and potentially risky. Why? Because if I have, say, 10 different places in my code doing the exact same thing, then I have 10 different places to modify/fix/improve if there's an issue. So how can we solve for this conundrum? One thing that can help is using functions.
Syntax and code structure
The coding syntax for a function can vary depending on the language but in principal, they all operate very similarly: it's a block of code that's designed to be reused for a specific purpose. Here's an example function as it might appear in python:
def greet(name):
print("Space. The final frontier.")
print(f"Live long and prosper, {name}!")
greet("Bob")
There's a few minimal elements here:
- The
def
keyword tells python that we want to define a function. - The indented code (which in this case is a couple print statements) determines what the function actually does when it's run.
- Later, at the bottom, we finally use the function (a concept commonly referred to as "calling" the function)
The output upon execution, is a very simple response back from the python interpreter:
Space. The final frontier.
Live long and prosper, Bob!
Simple, right? Now, imagine if I needed to do this same task a hundred different times for a hundred different names? I could avoid a lot excessive coding because I'd just need to call the function (instead of typing out all of those print
statements over and over).
Summary
So to recap, there are two high-level steps to using functions in python:
- We define the function and build out what it should actually do. We use the
def
keyword which gives the function a name and sets expectations in terms of input. We also have various indented lines of code so it can actually do something when executed. - We later call the function and actually use the code. This call is performed just by specifying the function name and a set of parenthesis. For example:
greet("Bob")
. Once we do this, the computer knows to execute the steps that were specified in the function itself.
For more information, check out these resources:
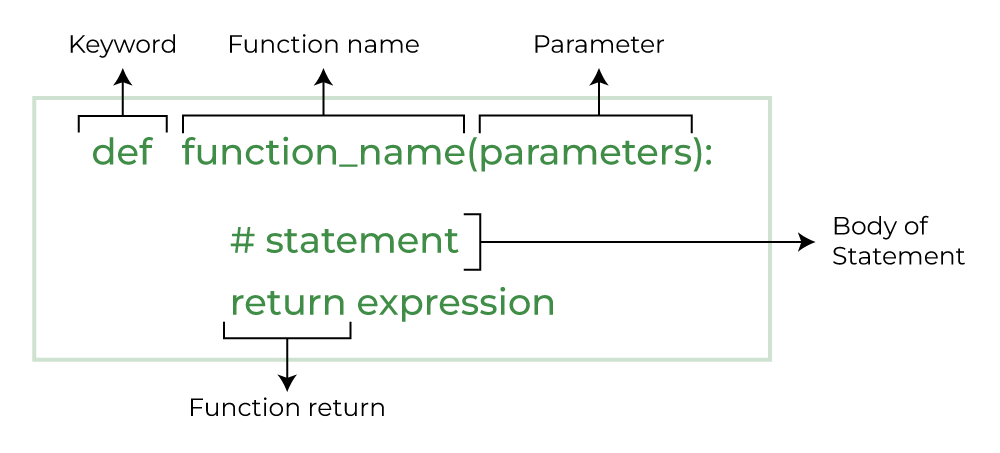
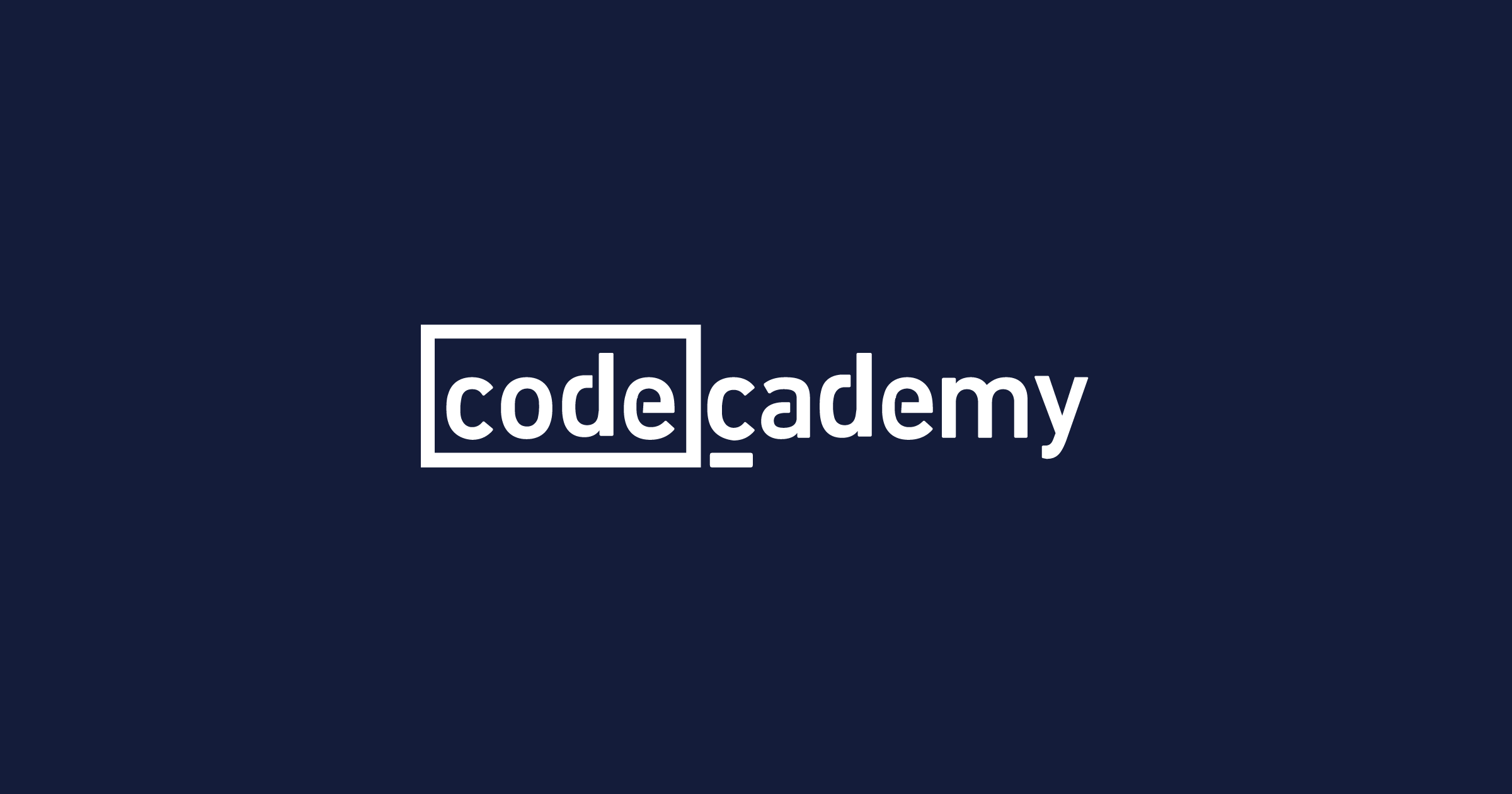
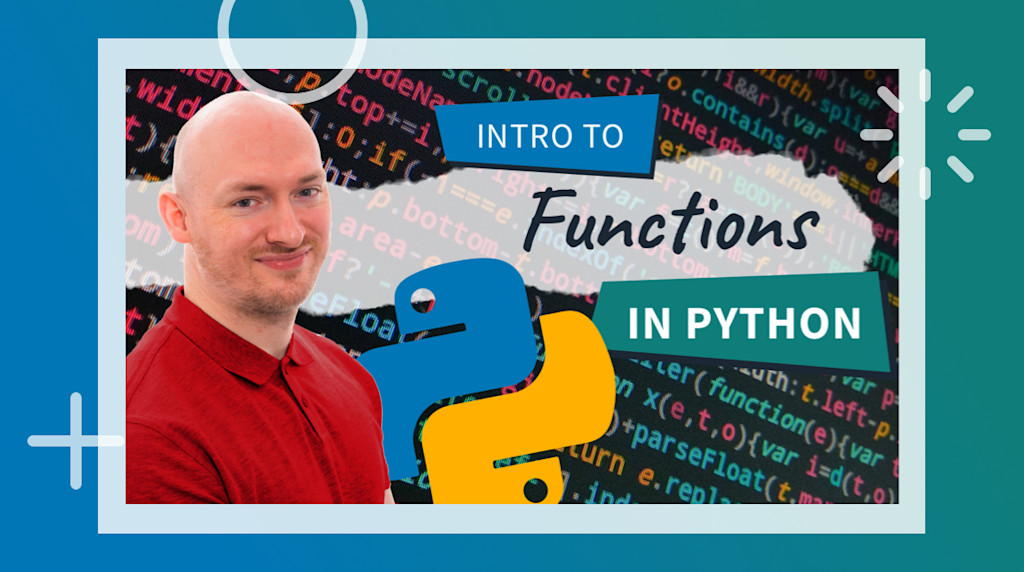